mybatis拦截器使用实战
定义注解
package com.zspearl.cloud.common.core.annotation;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
/**
* <p>Title: 注解类:mybatis自定义主键生成策略</p>
* <p>Description: </p>
* @author cwh
* @date 2022-11-16
*/
@Target(ElementType.FIELD)
@Retention(RetentionPolicy.RUNTIME)
public @interface AutoId {
String primarkey() default "";
IdType type() default IdType.SNOWFLAKE_ID;
enum IdType {
SNOWFLAKE_ID
}
}
package com.zspearl.cloud.common.core.annotation;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Target(ElementType.FIELD)
@Retention(RetentionPolicy.RUNTIME)
public @interface CreateBy {
}
package com.zspearl.cloud.common.core.annotation;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Target(ElementType.FIELD)
@Retention(RetentionPolicy.RUNTIME)
public @interface CreateTime {
}
package com.zspearl.cloud.common.core.annotation;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Target(ElementType.FIELD)
@Retention(RetentionPolicy.RUNTIME)
public @interface UpdateBy {
}
package com.zspearl.cloud.common.core.annotation;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Target(ElementType.FIELD)
@Retention(RetentionPolicy.RUNTIME)
public @interface UpdateTime {
}
拦截器实现
package com.zspearl.cloud.goldstreet.config.interceptor;
import com.zspearl.cloud.common.core.annotation.*;
import com.zspearl.cloud.common.core.utils.DateUtils;
import com.zspearl.cloud.common.core.utils.IdUtils;
import com.zspearl.cloud.common.core.utils.StringUtils;
import com.zspearl.cloud.common.security.service.TokenService;
import org.apache.ibatis.executor.Executor;
import org.apache.ibatis.mapping.MappedStatement;
import org.apache.ibatis.mapping.ParameterMap;
import org.apache.ibatis.mapping.SqlCommandType;
import org.apache.ibatis.plugin.*;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import org.springframework.util.ObjectUtils;
import org.springframework.util.ReflectionUtils;
import java.lang.reflect.Field;
import java.util.Date;
/**
* <p>Title: mybatis拦截器</p>
* <p>Description: </p>
* @author cwh
* @date 2022.11.16 10:56
*/
@Component
@Intercepts(@Signature(type = Executor.class,method = "update",args = {MappedStatement.class,Object.class}))
public class MybatisInterceptor implements Interceptor {
private Logger logger = LoggerFactory.getLogger(MybatisInterceptor.class);
@Override
public Object intercept(Invocation invocation) throws Throwable
{
/获取登录用户id
Long userId = *********;
if (StringUtils.isNull(userId))
{
userId = -1L;
}
Object[] args = invocation.getArgs();
MappedStatement arg = (MappedStatement) args[0];
if (SqlCommandType.INSERT.name().equals(arg.getSqlCommandType().name()))
{
ParameterMap parameter = arg.getParameterMap();
Class<?> entityClass = parameter.getType();
if (StringUtils.isNull(entityClass))
{
return invocation.proceed();
}
Long finalUserId = userId;
ReflectionUtils.doWithFields(entityClass, field->{
ReflectionUtils.makeAccessible(field);
AutoId annotation = field.getAnnotation(AutoId.class);
CreateTime createTime = field.getAnnotation(CreateTime.class);
CreateBy createBy = field.getAnnotation(CreateBy.class);
if (!ObjectUtils.isEmpty(annotation) && field.getType().isAssignableFrom(Long.class))
{
field.setAccessible(true);
Object value = field.get(args[1]);
if (StringUtils.isNotNull(value)) {
return;
}
setField(args[1],field, generateId(annotation.type()));
}
else if (!ObjectUtils.isEmpty(createTime) && field.getType().isAssignableFrom(Date.class))
{
setField(args[1], field, DateUtils.getNowDate());
}
else if (!ObjectUtils.isEmpty(createBy))
{
if (field.getType().isAssignableFrom(String.class))
{
setField(args[1], field, String.valueOf(finalUserId));
}
else if (field.getType().isAssignableFrom(Long.class))
{
setField(args[1], field, finalUserId);
}
}
});
}
else if (SqlCommandType.UPDATE.name().equals(arg.getSqlCommandType().name()))
{
ParameterMap parameter = arg.getParameterMap();
Class<?> entityClass = parameter.getType();
if (StringUtils.isNull(entityClass))
{
return invocation.proceed();
}
Long finalUserId = userId;
ReflectionUtils.doWithFields(entityClass, field->{
ReflectionUtils.makeAccessible(field);
if (!ObjectUtils.isEmpty(field.getAnnotation(UpdateTime.class)))
{
setField(args[1], field, DateUtils.getNowDate());
}
if (!ObjectUtils.isEmpty(field.getAnnotation(UpdateBy.class)))
{
if (field.getType().isAssignableFrom(Long.class))
{
setField(args[1], field, finalUserId);
}
else if (field.getType().isAssignableFrom(String.class))
{
setField(args[1], field, String.valueOf(finalUserId));
}
}
});
}
return invocation.proceed();
}
@Override
public Object plugin(Object target)
{
return target instanceof Executor ? Plugin.wrap(target, this) : target;
}
protected Object generateId(AutoId.IdType type)
{
Object id = null;
switch (type)
{
case SNOWFLAKE_ID:
//雪花ID生成
id = *****;
break;
}
return id;
}
protected void setField(Object target, Field field, Object attributeValue) throws IllegalAccessException
{
field.setAccessible(true);
field.set(target,attributeValue);
}
}
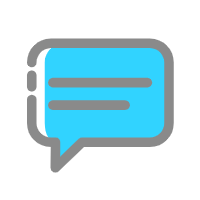
0 评论